C Programs
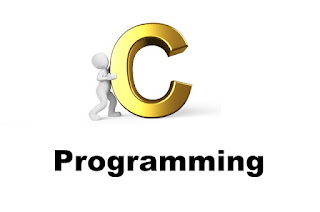
{tocify} $title= {Table
of Contents}
C Programs
C Programs
- Top 10+ C Programs
- Fibonacci Series
- Prime Number
- Palindrome Number
- C program to compare the two strings
- Strings Concatenation in C
- Factorial
- Armstrong Number
- Sum of Digits in C Program
- Count the number of digits in C
- Reverse Number
- Swap Number
- Print "Hello" without ;
- Assembly code in C
- C program without main
- Calculate Simple Interest in C Program
- Matrix Multiplication
- Decimal to Binary
- Number in Characters
- Alphabet Triangle
- Number Triangle
- Fibonacci Triangle
- Hexadecimal to Binary
- Hexadecimal to Decimal
- Octal to Hexadecimal in C
- Strong number in C
- Star Program in C
- itoa Function in C
- Extra Long Factorials in C
- Leap year program in C
- Perfect Number Program in C
- Variables vs Constants
- Round Robin Program in C with OutputC
- Program to find the roots of quadratic equation
- Type Casting vs Type Conversion
- How to run a C program in Visual Studio Code
- Modulus Operator in C/C++
- Sum of first N natural numbers in C
- Big O Notation in C
- LCM of two numbers in C
- while loop vs do-while loop in C
- Memory Layout in C
- Balanced Parenthesis in C
- Binary to Decimal Number in C
- GCD of two numbers in C
- Getchar() function in C
- flowchart in C
- Simpson Method
- Pyramid Patterns in C
- Random Function in C
- Floyd's Triangle in C
- C Header Files
- abs() function in C
- Atoi() function in C
- Structure Pointer in C
- sprintf() in C
- Range of Int in C
- C Program to convert 24 Hour time to 12 Hour time
- What is double in C
- What is the main in C
- Calculator Program in C
- Calloc in C
- user-defined vs library function in C
- Memset
- CASCII Table in C
- Static function in C
- Reverse a String in C
- Twin Prime Numbers in C
- strchr() function in C
- Structure of a C program
- Power Function in C
- Malloc in C
- Table Program in C
- Types of Recursion in C
- Convert Uppercase to Lowercase in C
- Unary Operator in C
- Arithmetic Operator in C
- Ceil Function in C
- Relational Operator in C
- Assignment Operator in C
- Pre-increment and Post-increment Operator in C
- Pointer vs array in C
- Restrict keyword in C
- The exit() function in C
- Const Qualifier in C
- Sequence Points in C
- Anagram in C
- Increment and Decrement Operators in C
- Logical AND Operator in C
- Shift Operators in C
- Near, Far, and Huge pointers in C language
- Magic Number in C
- Remove Duplicate Elements from an Array in C
- Generic Linked list in C
- isalnum() function in C
- isalpha() function in C
- Bisection Method in C
- snprintf() function in C
- Remove an element from an array in C
- Square Root in C
- isprint() function in C
- isdigit() function in C
- isgraph() function in C
- Logical NOT (!) Operator in C
- Self-referential structure
- Break Vs. Continue in C
- For vs. While loop in C
- Abort() Function in C
- Assert in C
- Floor() Function in C
- memcmp() in C
- Find Day from Day in C without Using Function
- Find Median of 1D Array Using Functions in C
- Find Reverse of an Array in C Using Functions
- Find Occurrence of Substring in C using Function
- Find out Power without Using POW Function in C
- Exponential() in C
- Float in C
- islower() in C
- memcpy() in C
- memmove() in C
- Matrix Calculator in C
- Bank Account Management System
- Library Management System in C
- 8th Sep - Calendar Application in C
- 8th Sep - va_start() in C programming
- 8th Sep - Ascii vs Unicode
- Student Record System in C
- Contact Management System in C
- Cricket Score Sheet in C
- C/C++ Tricky Programs
- Clearing the Input Buffer in C/C++
- In-place Conversion of Sorted DLL to Balanced BST
- Merge Two Balanced Binary Search Trees
- Responsive Images in Bootstrap with Examples
- Why can't a Priority Queue Wrap around like an Ordinary Queue
- Customer Billing System in C
- Builtin functions of GCC compiler
- Integer Promotions in C
- Bit Fields in C
- Department Management System in C
- Local Labels in C
- School Billing System in C
- Banking Account System in C using File handling
- Data Structures and Algorithms in C - Set 1
- Data Structures and Algorithms in C - Set 2
- Employee Record System in C
- Hangman Game in C
- Hospital Management System in C
- Number of even and odd numbers in a given rangeqsort() in C
- Quiz game in C
- An Array of Strings in C
- Peak element in the Array in C
- Move all negative elements to one side of an Array-C
- Reverse an Array in C
- 3 way merge sort in C
- Segregate 0s and 1s in an array
- Articulation Points and Bridges
- Execution of printf with ++ Operators
- Understanding the extern Keyword in C
- Banker's Algorithm in C
- FIFO Page Replacement Algorithm in C
- Kruskal's Algorithm in C
- FCFS Program in C
- Triple DES Algorithm in C
- Window Sliding Technique in C
- Sleeping Barber Problem Solution in C
- How to Add Matrix in C
- C Program to Demonstrate fork() and pipe()
- Deadlock Prevention using Banker's Algorithm in C
- Simple Stopwatch Program in C
- Add Node to Linked List in C
- How to Add two Array in C
- Algorithm in C language
- Add Digits of Number in C
- Add Element in Array in C
- Add String in C
- Add Two Matrices in C
- Add two numbers using Pointer in C