Math Functions in C Programming Languages
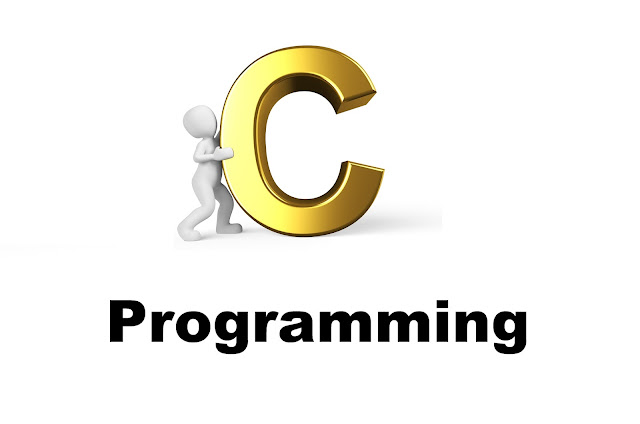
Introduction to Math Functions in C Programming Languages
This article
lists the different mathematical functions used in C programming languages with
working code illustration. Computers do huge mathematical calculations and
analyses of huge numbers, to do so we have used math features in C. Before
Starting with, we need to know the C languages use header/library called Math.h
for various mathematical functions. This helps in calculating trigonometric
operations, logarithms, absolute values, square roots. So, let us explore the
different types of functions used in this library. All these functions take
double as a data type and return the same. Math functions and constants are
defined in the C library, in <math.h> header file, which can be used to
perform mathematical calculations. C Programming allows us to perform
mathematical operations through the functions defined in <math.h> header
file. The <math.h> header file contains various methods for performing
mathematical operations such as sqrt(), pow(), ceil(), floor() etc.
The math.h header defines various C mathematical functions. All the functions available in this library take double as an argument and return double as the result. Let us discuss some important C math functions one by one.
Math Functions in C Programming Languages
There are
various methods in math.h header file. The commonly used functions of math.h
header file are given below.
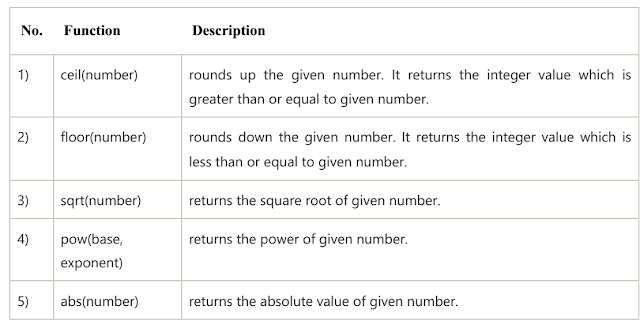
Various Math Functions in C
Let’s see various functions defined in math.h and the Math library is categorized into three main types: Trigonometric functions, math functions, Log/expo functions. To implement the below functions, it is mandatory to include<cmath.h> or <math.h> in the code.
1. floor (double a)
This
function returns the largest integer value not greater than ‘a’ value. It
rounds a value and returns a double as a result. It behaves differently for
negative numbers, as they round to the next negative number.
Ex: floor
(7.2) is 7.0
floor (-7.2) is -8.0
Example:
This program illustrates how to compute the floor for the declared value and rounds to the next value 10.
Programs of Floor Functions
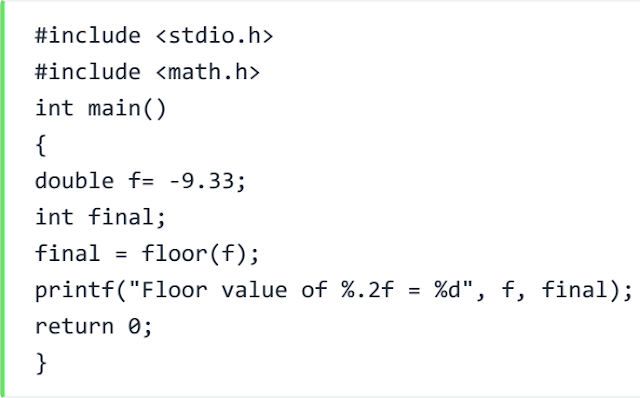
Output of Floor Functions

2. ceil ()
Syntax:
double ceil (double b)
This function returns the smallest integer value that is greater or equal to b and rounds the value upwards. For a negative value, it moves towards the left. Example 3.4 returns -3 has the output.
Example:
This program
explains by taking input in the float argument and returns the ceil value.
Programs of Ceil Functions
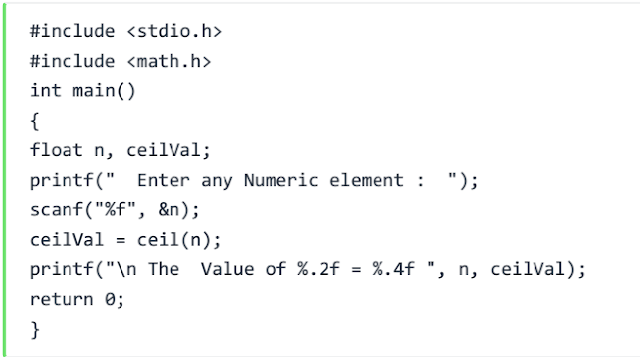
Output of Ceil Functions
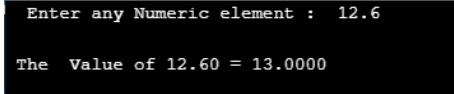
3.
Sqrt ()
The C library function double sqrt(double x) returns the
square root of x.
Syntax
double sqrt(double x);
Example:
Programs of sqrt()
Functions
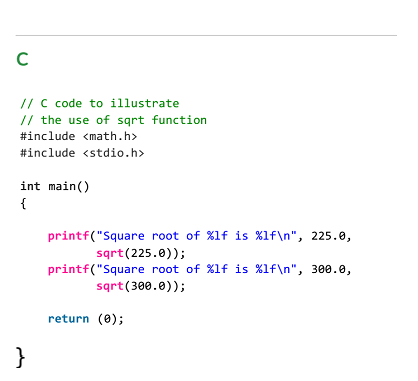
Output of sqrt() Functions
Square root of 225.000000 is 15.000000
Square root of 300.000000 is 17.320508
4.
round ()
This function rounds the nearest value of a given input. It
throws out the error if the value is too large. Other functions like lround (),
llround () also rounds the nearest integer.
Syntax:
intround(
arg
)
Example:
Programs of round() Functions
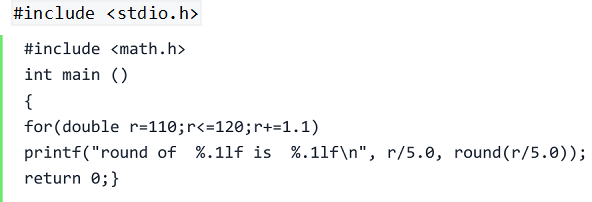
Output of round() Functions
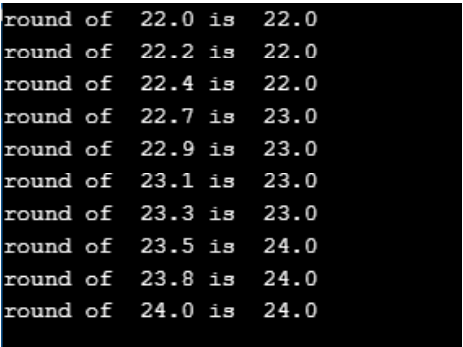
The below code is very simple which does round off to the nearest ‘r’ value in the for loop.
5. pow ()
The C
library function double pow(double x, double y) returns x raised to the power
of y
i.e. xy.
Syntax
double pow(double x, double y);
Example
Programs of pow()
Functions
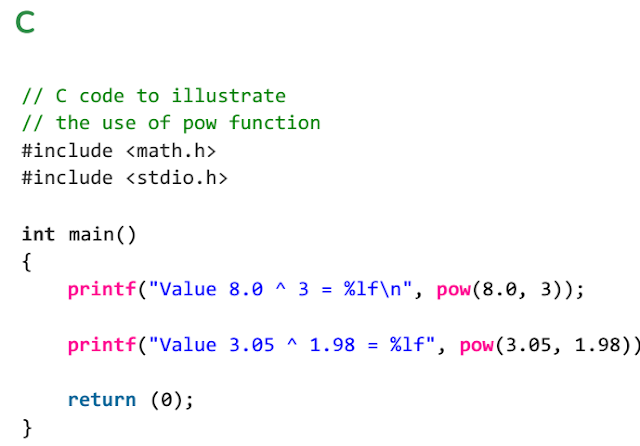
Output of pow() Functions
Value 8.0 ^
3 = 512.000000
Value 3.05 ^
1.98 = 9.097324
6. fabs()
The C library function double fabs(double x)
returns the absolute value of x.
Syntax
syntax: double fabs(double x)
Example
Programs of fabs()
Functions
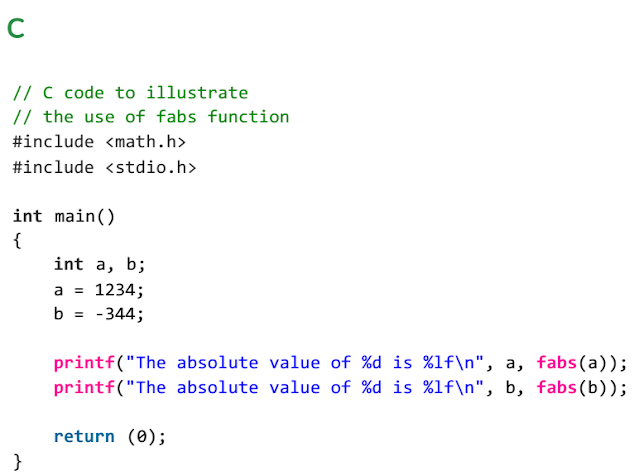%20Functions.png)
Output of fabs()
Functions
The absolute value of 1234 is 1234.000000
The absolute value of -344 is 344.000000
7. trun()
This
function helps in truncating the given value. It returns integer values. To
truncate floating and double values truncf (), truncl () are used.
Syntax:
doubletrunc(
a
);
Example:
Below source code takes two input values a, b to truncate the double values.
Programs of trun()
Functions
#include <stdio.h>
#include <math.h>
void main() {
double m, n, a, b;
a = 56.16;
b = 85.74;
m = trunc(a);
n = trunc(b);
printf("The value of
a: %lf\n",m);
printf("The value of
a: %lf\n",n);
}
Output of trun()
Functions

8. fmod()
This
function returns the remainder for the given two input values when m divided by
n.
Syntax:
doublefmod(double
I
,double
j
)
Example:
In the below example it takes two values from the user to compute the remainder using fmod() function.
Program of fmod()
Functions
#include<stdio.h>
#include<math.h>
intmain()
{
doublefiN
;
doublesecN
;
doublen
;
printf("Enter the first number: ");
scanf("%lf",&fiN
);
printf("Enter the second number: ");
scanf("%lf",&secN
);
printf("fmod(firstNumber,secondNumber) is %lf \n",fmod(fiN
,secN
));
}
Output of fmod() Functions
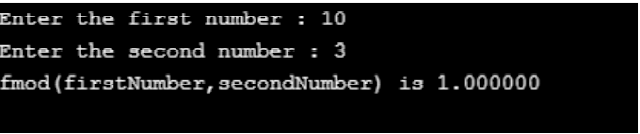
Trigonometric Functions
Below are
the different functions of Trigonometric:
1) sin()
This
built-in function gives sine value of the given number, calculates
floating-point values. asin() computes arc, for hyperbolic it is sinh().
Syntax:
returntype
sin(y
);
y returns
value in radians and return type takes double.
Example:
In the following source code, I have taken two different input values to calculate sin value and returns double.
Program of sin()
Functions
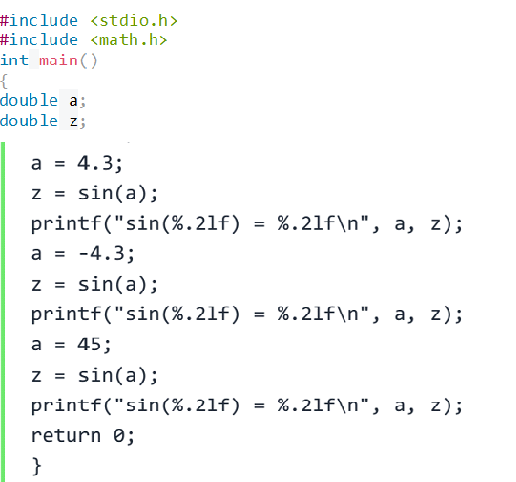.Program%20of%20sin%20Function.png)
Output of sin() Functions
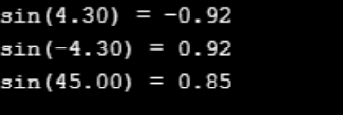.Output%20of%20sin%20Function.png)
2) cos()
This math
function determines the trigonometric cosine value for the given element.
Syntax:
return type cos(argument);
Example
Programs of cos() Functions
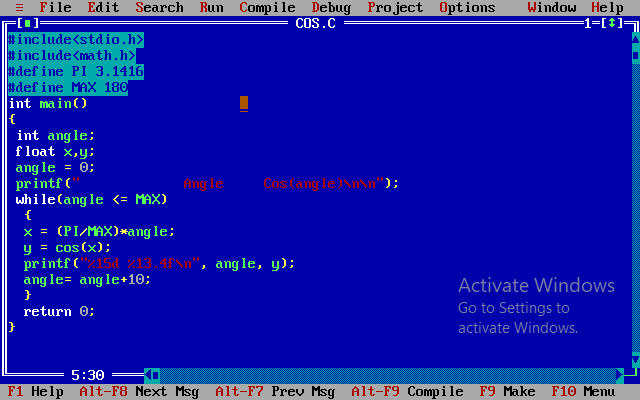.Program%20of%20Example%20of%20Cos%20Programs.png)
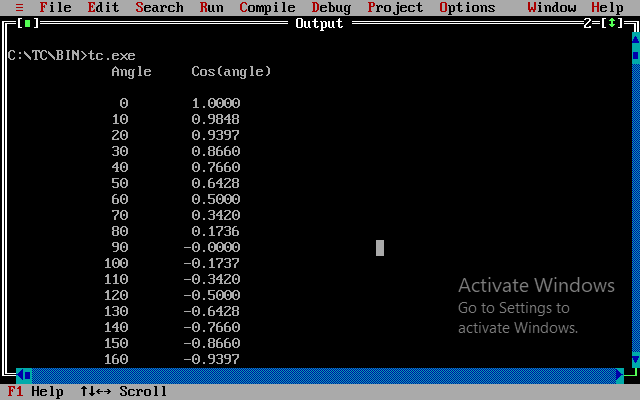.Output%20of%20Example%20of%20Cos%20Programs.png)
3) tan()
This math
library function calculates tangent values of the angle for the mathematical
expression and measured in radians.
It can be
declared as
doubletan(
arguments
);
Example
In the
following source code, tan value is calculated for the following angles which
is incremented using for loop.
Program of tan() Functions
# include <stdio.h>
# include <conio.h>
# include <math.h>
void main()
{
float z;
int k;
char ch;
printf("\nAngle \t Tan
\n");
for(k = 0; k <= 180; k = k + 30)
{
z = k * 3.14159 / 180;
printf("\n %d, %5.2f”, k, tan(z));
}
getch();
}
Output of tan() Functions
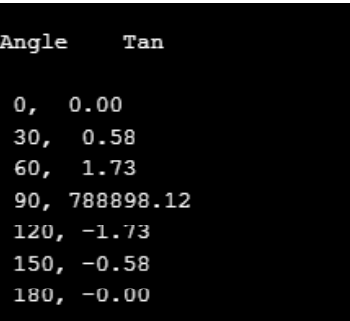.Output%20of%20tan%20Function.png)
Log Arithmetic Functions
Below are
the different functions of log arithmetic:
a).
exp()
This
function does computation on exponential for a given value(ex). There are also
other subtypes like frexp(), Idexp() returning mantissa and multiplied to the
power of x.
Syntax:
returntype
exp(value
);
Example:
The program
takes numeric value from the user to compute the exponent for a given value and
returns double.
Program of exp() Functions
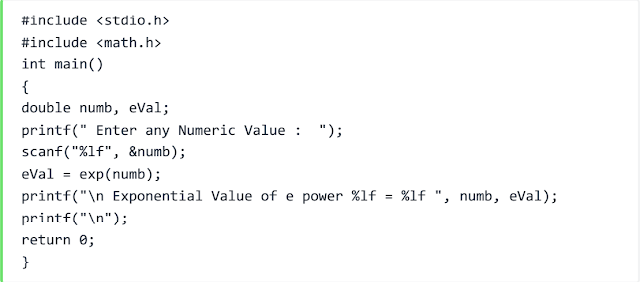.Program%20of%20exp%20Function.png)
Output of exp() Functions
.Output%20of%20Exp%20Function.png)
b) .
log()
This
function returns the logarithm value of a given number. (to the base e. loge)
Syntax:
doublelog(
arg
);
Example:
In the
following example, log value for the given number is calculated using function.
User-defined function lgm() does computation and function is called in the main
function.
Program of log() Functions
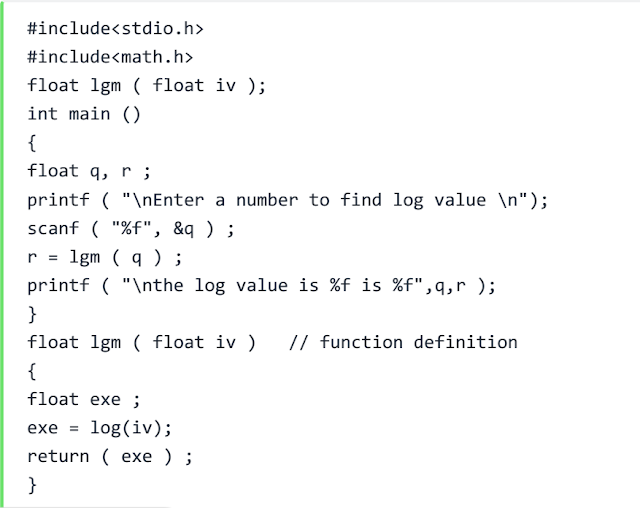.Program%20of%20log%20Function.png)
Output of log() Functions
.Output%20of%20log%20Function.png)
Example of Math Functions in C Program
Let's see a simple example of math functions found in math.h header file.
Programs of Example of Math Functions in C Program
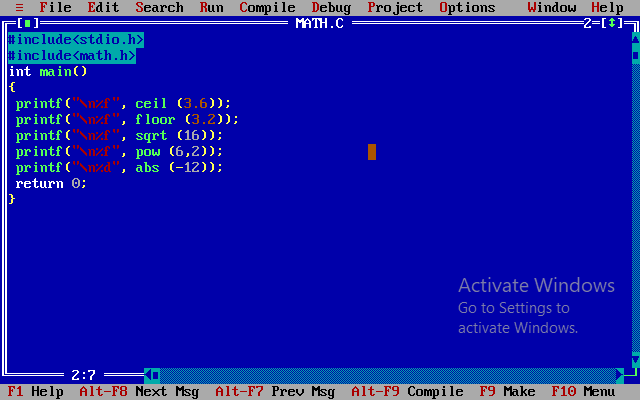
Output of Example of Math Functions in C Program
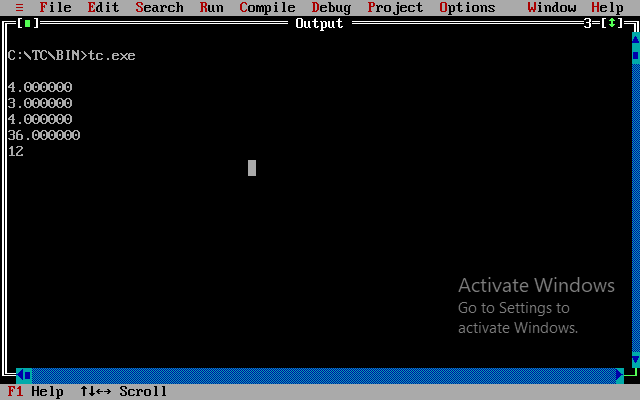